Coding Solution - Linked List Cycle
- Admin
- Feb 11, 2022
- 1 min read
This is similar to the Leetcode Problem - (11) Linked List Cycle - LeetCode
Given head, the head of a linked list, determine if the linked list has a cycle in it.
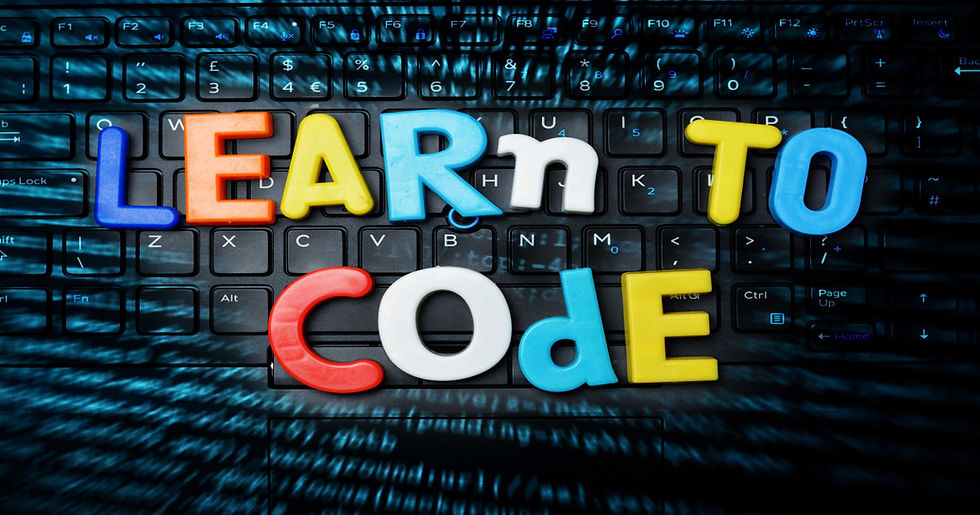
The approach would be simple, take 2 pointers, slow as turtle and fast as hare. When the turtle is equal to the hare, cycle is found.
Do have a look at the discuss section for more optimized solutions if available - (11) Linked List Cycle - LeetCode Discuss
/**
* Definition for singly-linked list.
* class ListNode {
* int val;
* ListNode next;
* ListNode(int x) {
* val = x;
* next = null;
* }
* }
*/
public class Solution {
public boolean hasCycle(ListNode head) {
ListNode hare = head;
ListNode turtle = head;
while(hare!=null && hare.next !=null) {
turtle = turtle.next ;
hare = hare.next.next;
if(hare == turtle)
return true;
}
return false;
}
}
Comments