Coding Solution - Linked List Cycle with Node at intersection
- Admin
- Feb 11, 2022
- 1 min read
This is similar to the Leetcode Problem -(11) Linked List Cycle II - LeetCode
Given the head of a linked list, return the node where the cycle begins. If there is no cycle, return null.
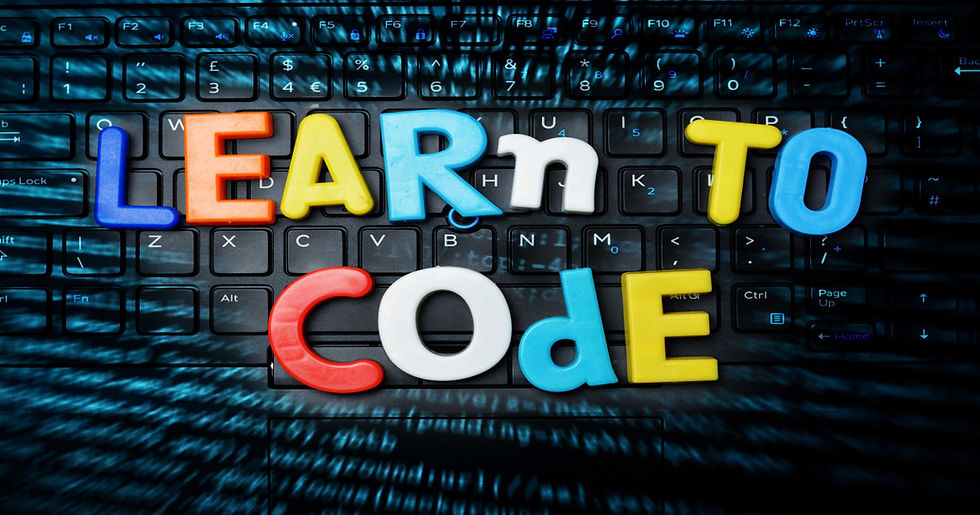
The approach would be similar to linked list cycle, take 2 pointers, slow as turtle and fast as hare. When the turtle is equal to the hare, cycle is found. Once cycle is found, slow will start from head. Both slow and fast will traverse and when they are equal again, that node is the start of the cycle
Do have a look at the discuss section for more optimized solutions if available - (11) Linked List Cycle II - LeetCode Discuss
/**
* Definition for singly-linked list.
* class ListNode {
* int val;
* ListNode next;
* ListNode(int x) {
* val = x;
* next = null;
* }
* }
*/
public class Solution {
public ListNode detectCycle(ListNode head) {
ListNode slow = head, fast = head;
while(fast!=null && fast.next !=null) {
slow = slow.next;
fast = fast.next.next;
if(slow == fast) {
slow = head;
while (slow!=fast) {
slow = slow.next;
fast = fast.next;
}
return slow;
}
}
return null;
}
}
Comments