Coding Solution - Reverse a Linked List
- Admin
- Feb 9, 2022
- 1 min read
Updated: Feb 13, 2022
This is similar to the Leetcode Problem - (11) Reverse Linked List - LeetCode
Given the head of a singly linked list, reverse the list, and return the reversed list.
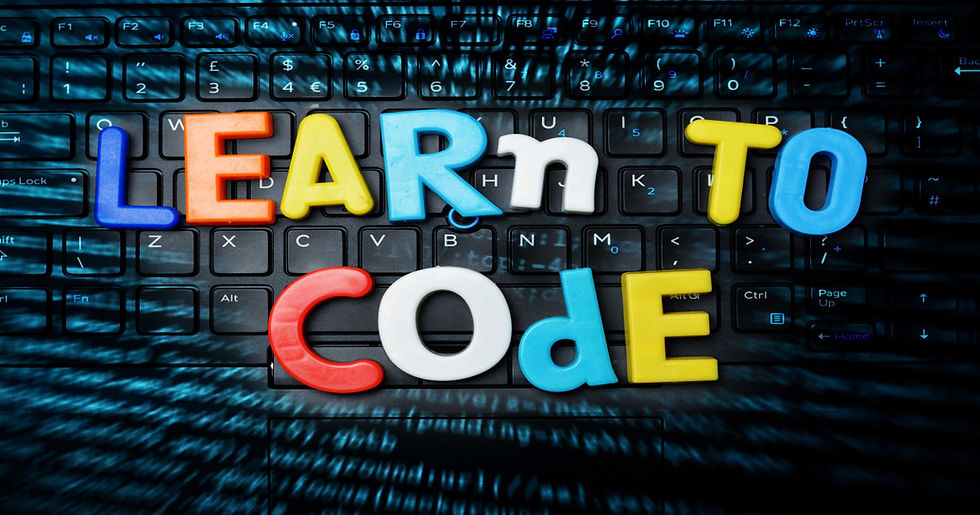
The approach would be simple, create a null node, place it to the left or first node and then reverse all the nodes from the right towards the null node.
Do have a look at the discuss section for more optimized solutions if available - (11) Reverse Linked List - LeetCode Discuss
/**
* Definition for singly-linked list.
* public class ListNode {
* int val;
* ListNode next;
* ListNode() {}
* ListNode(int val) { this.val = val; }
* ListNode(int val, ListNode next) { this.val = val; this.next = next; }
* }
*/
class Solution {
public ListNode reverseList(ListNode head) {
if(head ==null || head.next ==null)
return head;
ListNode newHead = null;
while (head!=null) {
ListNode next = head.next;
head.next = newHead;
newHead = head;
head = next;
}
return newHead;
}
}
Comentários